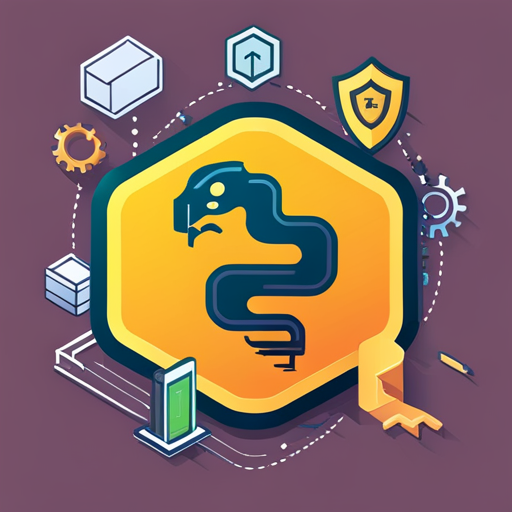
Hey there, intrepid coder! π Ever felt the itch to explore the vast universe of AWS (Amazon Web Services)? Whether youβre a seasoned developer or just starting out, if you’re into Java or Python (or both!), we’ve got a treat in store for you! Dive in as we unravel the magic of AWS development.
π Setting The Scene: What’s AWS?
Think of AWS as a massive digital playground. From computing power to storage solutions to machine learning, AWS offers over 200 services! It’s the Swiss Army knife of the cloud universe.
π Why Should Java & Python Devs Jump into AWS Development?
- Mammoth Library Support: AWS SDKs for both Java and Python are incredibly robust.
- Versatility: Whether you’re into web apps, data processing, AI, or IoT, AWS has a service waiting for you.
- Scalability & Flexibility: AWS handles the heavy lifting, allowing you to scale effortlessly.
π¨ AWS Essentials for Java & Python Devs: A Quick Tour
- Amazon EC2 (Elastic Compute Cloud): Virtual servers in the cloud. Think of them as your digital workstations.
- Java Example: Deploy a Spring Boot app on EC2.
- Python Example: Host a Flask/Django web application.
- Amazon S3 (Simple Storage Service): A place to store files and data. Imagine an infinite digital warehouse.
- Java/Python Use Case: Store user-uploaded images or files for your web application.
- AWS Lambda: Run your code without managing servers.
- Java Example: Process data from DynamoDB triggers.
- Python Example: Automatically generate thumbnails for new images uploaded to S3.
- Amazon DynamoDB: A NoSQL database service.
- Java/Python Use Case: Store user profiles or application settings.
β¨ AWS Development: Dive into Examples
- Python & AWS Lambda:
- Scenario: Resize images uploaded to S3.
import boto3
s3 = boto3.client('s3')
def lambda_handler(event, context):
# Get the file details
bucket = event['Records'][0]['s3']['bucket']['name']
key = event['Records'][0]['s3']['object']['key']
# Use a Python library like Pillow to resize
# ...
# Save the resized image back to S3
s3.put_object(Bucket=bucket, Key=key, Body=resized_image)
return "Image resized!"
Deploy this to AWS Lambda and let it listen to S3 upload events.
Java & DynamoDB:
- Scenario: Store and retrieve user data.
AmazonDynamoDB client = AmazonDynamoDBClient.builder().build();
DynamoDB dynamoDB = new DynamoDB(client);
Table table = dynamoDB.getTable("Users");
// Add a new user
Item user = new Item().withPrimaryKey("UserID", "12345").withString("Name", "John");
table.putItem(user);
// Retrieve a user
Item retrievedUser = table.getItem("UserID", "12345");
π Tips to Kickstart Your AWS Development Journey:
- Use the Free Tier: AWS offers a generous free tier. Experiment without burning a hole in your pocket.
- Stay Secure: AWS’s IAM (Identity and Access Management) is your guardian angel. Use it to manage access to your resources.
- Keep Learning: AWS is massive. Use resources like AWS’s documentation, online courses, and community forums.
Closing Notes
Alright, code wranglers, that was a swift cruise through the expansive AWS galaxy. Our introduction to AWS development aimed to ignite your curiosity and give you a taste of the wonders you can achieve with AWS, especially with Java and Python by your side.
Remember, in the world of AWS, the sky isn’t the limit; it’s just the beginning. Ready to explore further? Strap in, and let your AWS adventure begin!